So far in this book, every time that we created a variable, whether it was a String
, an Int
, or a Boolean
, we assigned a value to it. There are times, though, when we need to create a variable that might not actually hold a value!
This brings us to the exciting topic of nulls!
Introduction to Nulls in Kotlin
James has set up a coffee stand downtown, and he’s ready to start sharing his fine brew! After handing out each cup, he asks the guest to review the coffee, so that he can share the ratings with others who might be interested.
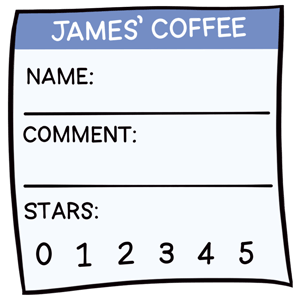
Since he wants to keep track of the ratings in a Kotlin program, he writes this simple class:
The name
is the name of the person who is reviewing his coffee, the comment
property is used for any comment that they want to share about it, and the stars
property holds the star rating - the number of stars that they give the coffee, between 0 and 5.
The first three guests of the day have filled out the review cards - let’s see how they rated his coffee!
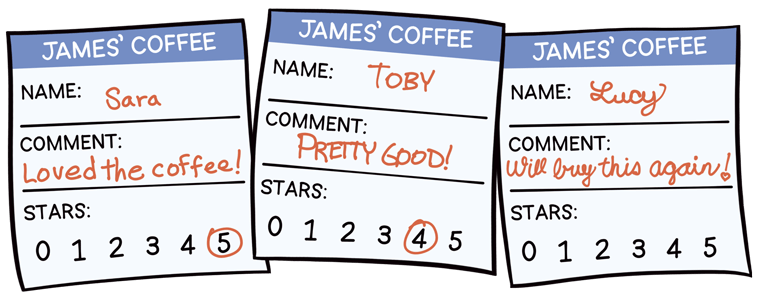
James instantiates some CoffeeReview
objects to record the three reviews that he received. He starts with the first two…
When he gets to Lucy’s review, though, he noticed that she forgot to leave a star rating. “That’s okay,” he said to himself, “I’ll just use the number zero since she didn’t mark any stars.”
He was ready to show the reviews on a screen, so he wrote a simple function, and called it with each of the reviews that he received:
This is what showed on the screen:
Latest coffee reviews --------------------- Sara gave it 5 stars! Toby gave it 4 stars! Lucy gave it 0 stars!
He thought that his solution would work well, but when the guests saw the review, they thought, “Wow, if Lucy didn’t like the coffee, maybe it’s not good. I’ll go somewhere else.”
Yikes!
James realized that a zero-star rating is not the same thing as having _no star rating~.
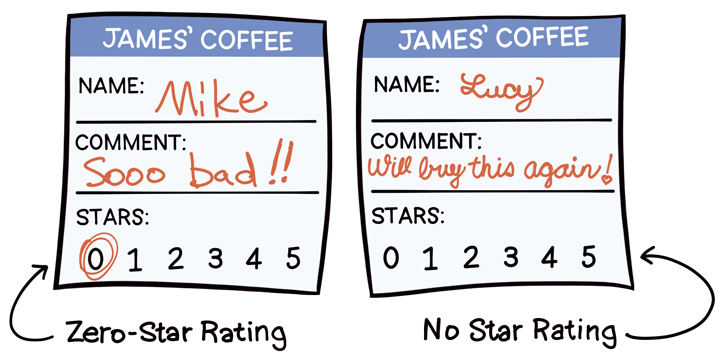
When someone doesn’t leave a star rating, then James doesn’t want to show a zero-star rating on the screen. Instead, he needs a way to tell Kotlin that they didn’t leave a star rating at all. How can he do that?
Present and Absent Values
As you might recall from Chapter 1, a variable is like a bucket that holds a value.
In all the code that we’ve written so far, we’ve created variables that contain a value. In other words, we’ve always had something inside that bucket. For example, a stars
bucket contains an Int
, like the number 5
:
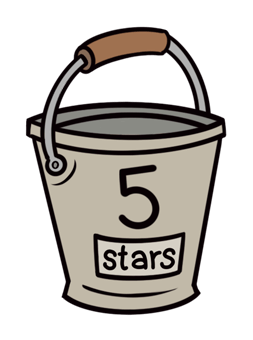
For the CoffeeReview
class, we need a stars
bucket that might or might not have a value in it. When the guest leaves a rating, the bucket needs to contain that value, but when they forget to rate it, that bucket needs to be empty.
So we want a bucket where we can either put a value inside of it, or leave it empty. This brings up two new terms:
- When a variable has a value inside of it, we’ll say that the value is present.
- When a variable does not have a value inside of it, we’ll say that the value is absent.
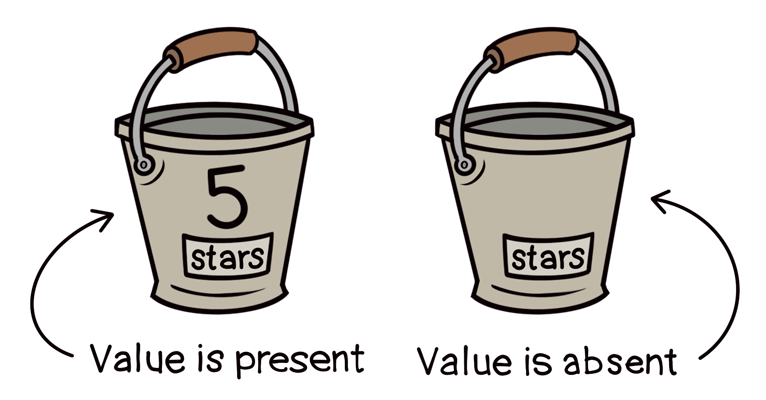
Kotlin uses a keyword called null
to represent the absence of a value.1 A variable that is assigned null
is like a bucket that is empty.2
For a review where there’s no star rating, we want to set stars
to null
. We can try giving it a null
when we construct Lucy’s CoffeeReview
, but when we do that, we get an error:
In fact, even apart from the CoffeeReview
class, if we simply create an Int
variable called stars
, we can’t assign null
to it.
Why is this?
In some programming languages, you can assign a null
to any variable. That might sound like a good idea, but it can result in lots of surprises while your code is running, because we never have any guarantees that the value of a variable is present.
In order to help prevent these kinds of problems, Kotlin won’t let you assign null
to just any variable. Instead, you have to clearly indicate when a variable can be empty.
How can we do this?
Nullable and Non-Nullable Types
In Kotlin, we use different types to indicate whether a variable can or cannot be set to null
.
All of the types that we’ve used so far in this book - such as String
, Int
, and Boolean
- require you to assign an actual value. You can’t just assign null
to them, as we tried above. Since they don’t let you assign null
, we call them non-nullable types. In contrast, types that allow you to assign null
are called nullable types.
In other words:
- When you want to guarantee that a variable’s value will be present - that is, when the value is required - then give the variable a non-nullable type.
- When you want to allow a variable’s value to be absent - that is, when the value is optional - then give the variable a nullable type.
In Kotlin, nullable types end with a question mark. For example, while Int
is a non-nullable type, Int?
(ending with a question mark) is a nullable type.
For every non-nullable type, a corresponding nullable type exists.
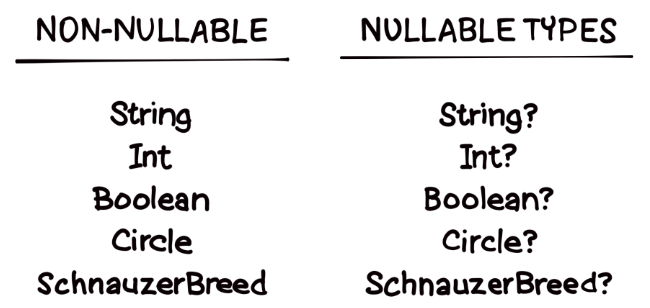
Let’s look at our code from Listing 6.6 again:
To allow this variable to accept a null, we simply change the type of the variable from the non-nullable type Int
to the nullable type Int?
, like this:
Now, stars
can be set to null
. Of course, it can also still be set to a normal integer value. For example:
Compile Time and Runtime
The type of a variable tells us whether that variable can hold a null, but it cannot tell us whether it actually does hold a null.
This brings up an important distinction to consider. There are things we can know when Kotlin is reading the code, and there are things we can know when we’re running the code.
- The point at which Kotlin is reading our code is called compile time. If we’re using an IDE like IntelliJ or Android Studio, this happens while we are writing the code.
- The point at which our computer runs our Kotlin code is called runtime.
You can think of compile time as the point when a plumber is assembling some water pipes, whereas runtime is like the point after someone has turned on the faucet, so water is running through those pipes.
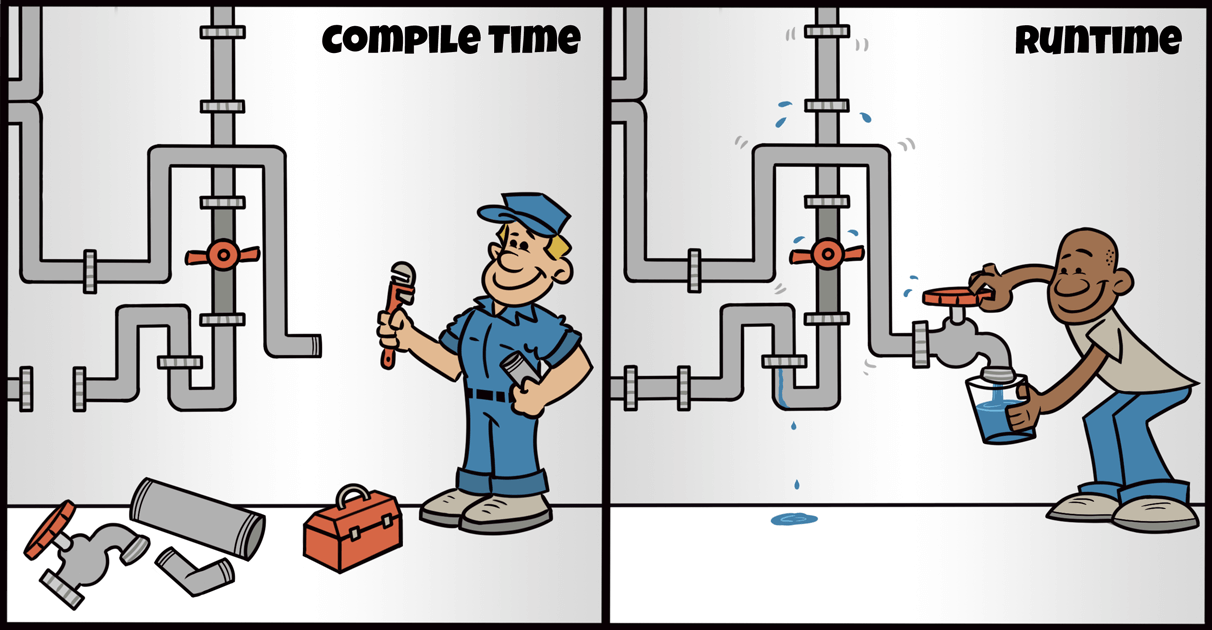
Kotlin knows the type of a variable at compile time, which is why it knows whether it can hold a null while we’re writing the code. On the other hand, Kotlin won’t know whether a variable actually holds a null until runtime.
In some simple cases like Listing 6.9, where we’re setting the variable with a literal directly in the code, it seems obvious to us whether the value is present or absent. In fact, your IDE (like IntelliJ or Android Studio) might even warn you when you use a non-null value with a variable that’s declared to be nullable - like saraStars
and tobyStars
above.
But values can also come from external sources, like databases, files on your hard drive, or when a user types on a keyboard. And once you start calling functions that have parameters, it’s possible that the value of the argument is absent when called from one place, and present when called from another.
In order to know whether a variable actually has a value at runtime, we have to convert it from a nullable type to a non-nullable type. We’ll see some cool tricks for this below! But first, it’s important to understand the relationship between nullable and non-nullable types.
How Nullable and Non-Nullable Types are Related
Even though Int
and Int?
are related, they’re still two different types, and you can’t just use an Int?
anywhere that you would use an Int
. For example, a function that expects an Int
won’t work if you try to send it an Int?
instead:
Why is this so?
To understand this, let’s turn our attention away from the review screen, and toward the front counter, where James is taking the coffee orders!
Expecting a Nullable Type
At the moment, James is running a non-profit coffee charity, which provides warm beverages to anyone, even if they can’t pay for it. If the guest would like to donate a payment, they can, but it’s not required.
Here’s a function that models this arrangement. The payment at a charity is optional, so we’ll make the payment
parameter nullable.
Naturally, if someone orders a coffee and provides payment, James gladly will give them a coffee!
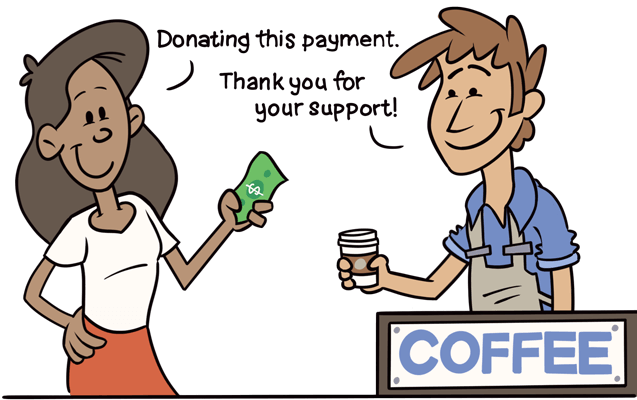
Passing a Payment
argument to the orderCoffee()
function is like this scenario - the guest is definitely providing payment.
Now, imagine that someone walks in and says, “This box might have a payment… or it might be empty. You can have whatever’s inside.” James says, “Even if it’s empty, that’s fine. We’re a charity, after all. Here’s your coffee!”
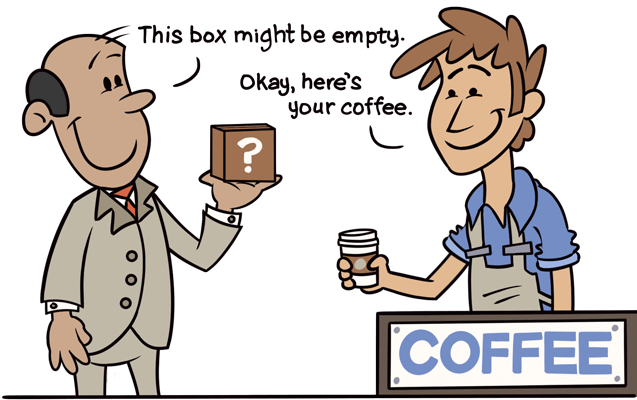
When we pass a Payment?
argument to the orderCoffee()
function, it’s like the guest is handing James a mystery box that contains either a payment or nothing at all.
To summarize, a function that has a nullable parameter like Payment?
is like a charity - it can accept an argument that has a non-nullable type (like Payment
), and it can also accept an argument that has a nullable type (like Payment?
). Either one is fine.
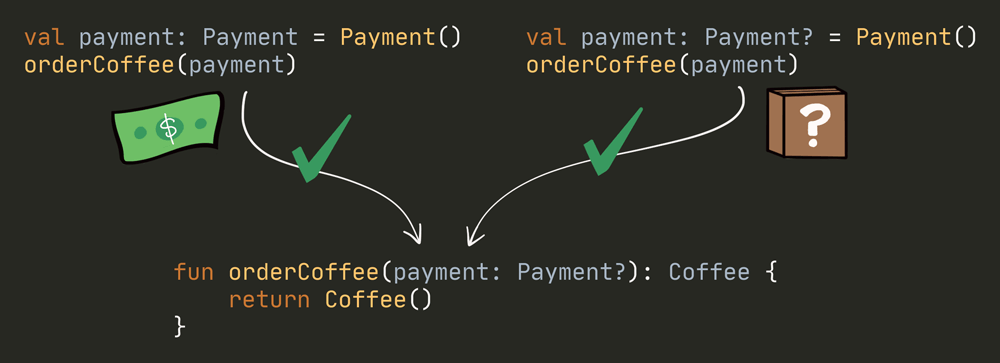
Expecting a Non-Nullable Type
After a while, James realized that he couldn’t get enough donations to sustain the charity, so now he’s running his coffee stand as a business. All those coffee beans cost money, and since the business doesn’t run from donations, he must receive payment in order to provide coffee to the customer.
Here’s a new version of orderCoffee()
that works like a business rather than a charity. Notice that the parameter has a non-nullable type, because payment
is now required.
As before, when someone orders a coffee and provides payment, James will gladly give them a coffee.
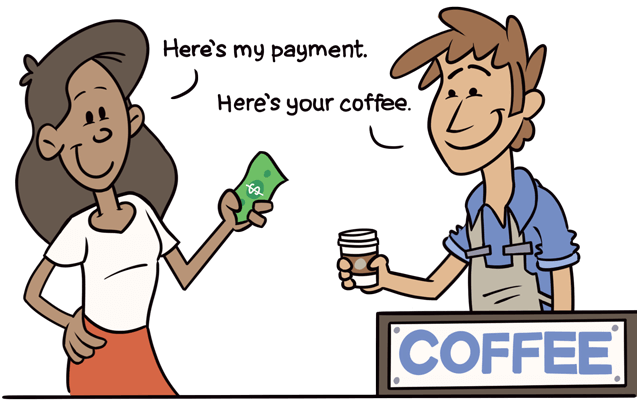
Passing a Payment
variable to this function is like this scenario - the guest is definitely providing payment, so everything works just fine.
Now imagine that someone orders a coffee, but instead of giving him payment, holds out a box, and says, “This box might have a payment… or it might be empty. I’ll trade you whatever’s inside this box for a coffee.”
“No deal!” he says. “You have to actually pay for your coffee! I can’t trade the coffee for a chance to receive payment. I have to actually receive payment!”
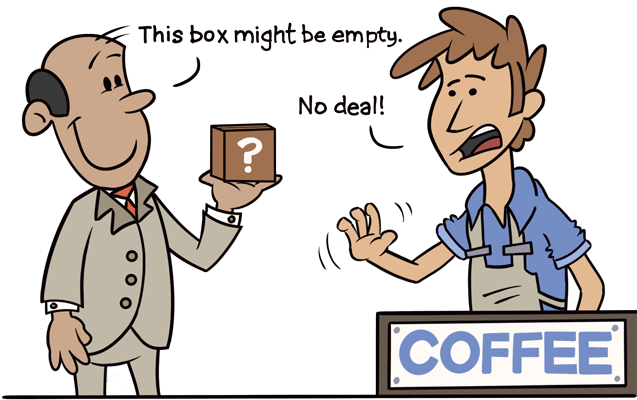
Passing a Payment?
variable to this function is like this scenario - the guest is either handing James a payment or nothing at all. Just like James, Kotlin says, “No deal!” (…well, actually it says, “Type mismatch.”)
When James requires payment, he can’t accept a payment that might not be there. It must be there. So a function that has a parameter of type Payment
is like a business - it cannot accept an argument of type Payment?
.
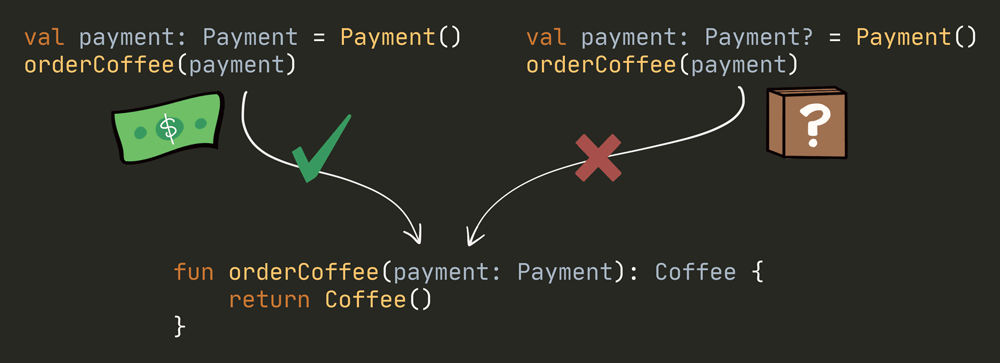
To summarize, you can use a non-nullable type (e.g., Payment
) where a nullable type (e.g., Payment?
) is expected, but not the other way around.
Now, it’s quite possible that the customer’s box actually has a payment inside! If only they would take the payment out of the box, then they could exchange that payment for coffee!
Similarly, Kotlin gives us a few different ways to safely convert a nullable type to a non-nullable type. Let’s take a look!
Using Conditionals to Check for null
Here again is the function for the coffee shop business:
When the customer tried to pay with a box that might be empty, it looked like this:
One very simple way to still order a coffee in this case is to check to see if payment
actually has a value when the code is running. In other words, we can look inside the box, and if the payment is not null
, then we can order the coffee.
There’s no error when you write this code, and orderCoffee()
will be called when you run it.
How does this work? Why can we call orderCoffee(payment)
in Listing 6.19 but not Listing 6.18?
Even though we declared payment
to be of type Payment?
(which is nullable), inside the if
block, its type changes to Payment
(which is non-nullable)! Kotlin knows that payment
must have a value inside that block, because we checked for it! This is called a smart cast.
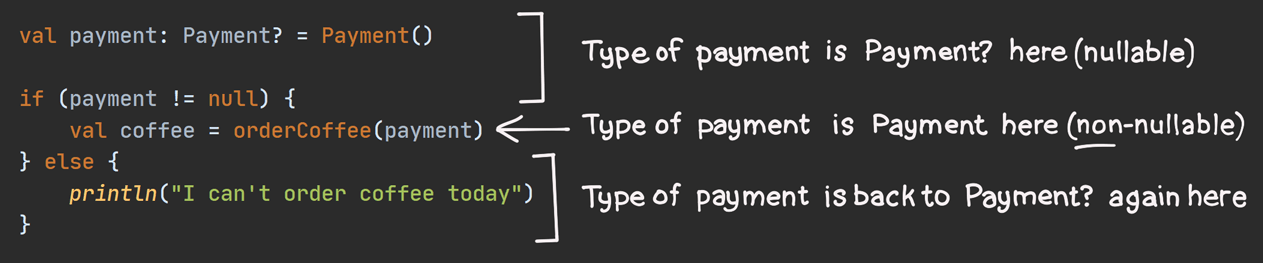
Smart casts also work with a when
conditional, like this:
By the way, this isn’t the only kind of smart cast that Kotlin can perform. We’ll see this again in a future chapter when we cover advanced object and class concepts.
So, using a conditional in this way is like opening the box, and if there’s something inside it, we order the coffee.
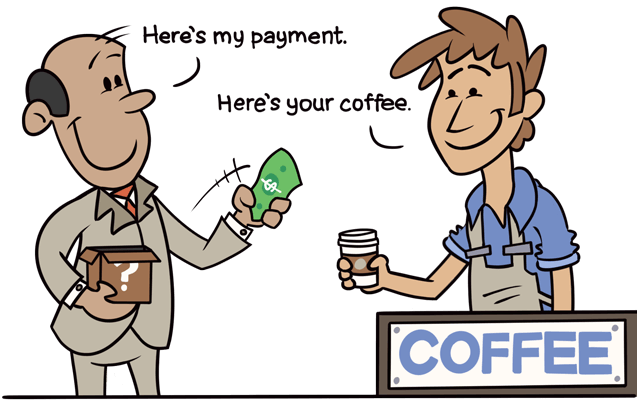
Otherwise, if there’s nothing inside the box, we don’t order the coffee.
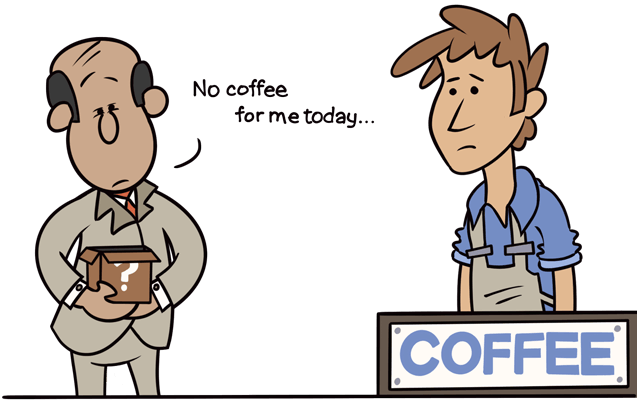
Using a conditional to do a smart cast is just one way to convert something that’s nullable to something that’s non-nullable! Next, let’s look at the elvis operator.
Using the Elvis Operator to Provide a Default Value
In the code above, we only ordered coffee when a value was present in the payment
variable. It sure would be nice if we could order a coffee even when we don’t have payment. For example, if our payment
variable is null, maybe our friend can pay for us!
This allows us to order coffee in either case. If payment
actually has a value, we can use that. Otherwise, we call the getPaymentFromFriend()
function, which returns a Payment
value that we can use instead.
As we learned back in chapter 3, instead of an if statement we can use an if expression, which pulls the coffee
variable outside of the if
and else
blocks. Let’s make that small change to our code, in order to make it more concise.
We’re also calling orderCoffee()
in both branches, so let’s pull that out of the if
and else
blocks, as well:
The code highlighted in Listing 6.23 is pretty common when dealing with nullable types: check whether a value is present… if so use that value, otherwise use some default value. To make this common expression easier, Kotlin gives us the elvis operator ?:
, so named because if you tip your head to the left and squint your eyes, it kind of looks like an emoticon of Elvis Presley’s hair line above a pair of eyes. (You might have to use your imagination a little!)
Anyway, here’s how we can use the elvis operator to make our code more concise:
This code works the same as the code in Listings 6.21, 6.22, and 6.23 - it’s just shorter and easier to read.
Using an elvis operator is like opening the box, and if there’s something inside it, we use that.
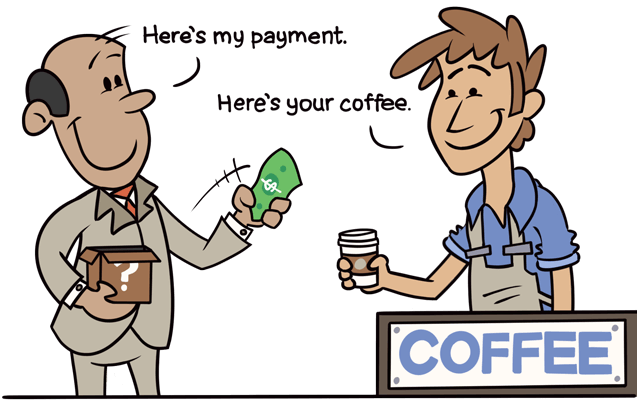
Otherwise, if the box is empty, we get a value from somewhere else and use that instead.
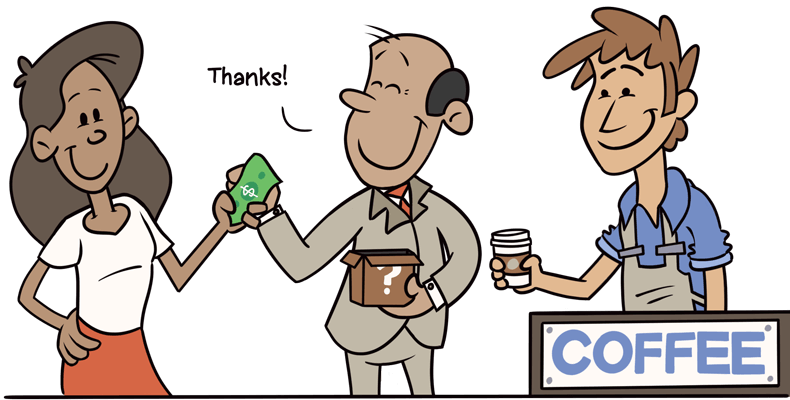
Using the Not-Null Assertion Operator to Insist that a Value is Present
I hesitate to mention this one. It’s dangerous, but in some rare cases, it can be a helpful option.
If you know for sure that a nullable variable will definitely have a value when your code is running, then you can use the not-null assertion operator !!
to evaluate it to a non-nullable type. Here’s how it would look when ordering coffee:
The type of the payment
variable is Payment?
, which is nullable, but the type of the expression payment!!
is Payment
, which is non-nullable.
By putting !!
after the variable name payment
, it’s like you’re saying to Kotlin, “Trust me… when the code runs, payment
will not be null!” If you’re wrong about that - if the variable is indeed null, you’ll get an error when the code runs:
The not-null assertion operator is like reaching into the box, and if there’s something inside, we use that.
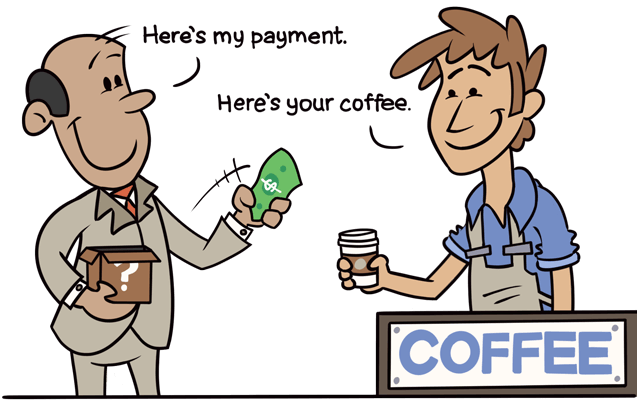
Otherwise, if the box is empty…
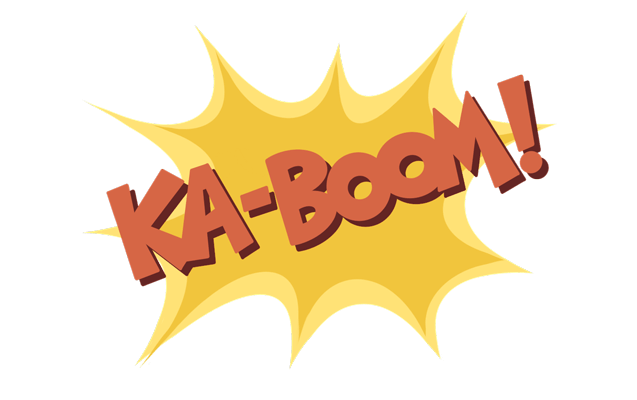
This is why the not-null assertion operator is dangerous! In the other cases above - using a conditional to check for null, and using an elvis operator - it wasn’t possible for us to get an error, because Kotlin’s rules about nullable types wouldn’t allow it. But here, we’re foregoing that null safety and taking on risk that the variable might actually be null when the code is running.
Compile-Time and Runtime Errors
We can get errors during either compile time or runtime.
- Listing 6.18 shows an example of a compile-time error - the IDE highlights the problem while we’re writing code.
- Listing 6.26 above shows code that will cause a runtime error, but unlike the compile-time error, there’s no highlight to let us know that an error will happen.
As a general rule, an error during compile time is more helpful than an error during runtime, because we know about it sooner. In fact, Kotlin won’t even let us run our code until we’ve fixed it! Runtime errors, on the other hand, are nefarious and they’re often more difficult to hunt down.
When we use the not-null assertion operator !!
, we’re avoiding a compile-time error, but taking a risk that we could end up with a runtime error. If you’re certain that the variable will not be null at runtime, then consider using a non-nullable type instead. If, for some reason, you can’t do that, then the not-null assertion operator might be what you need. But use it only as a last resort!
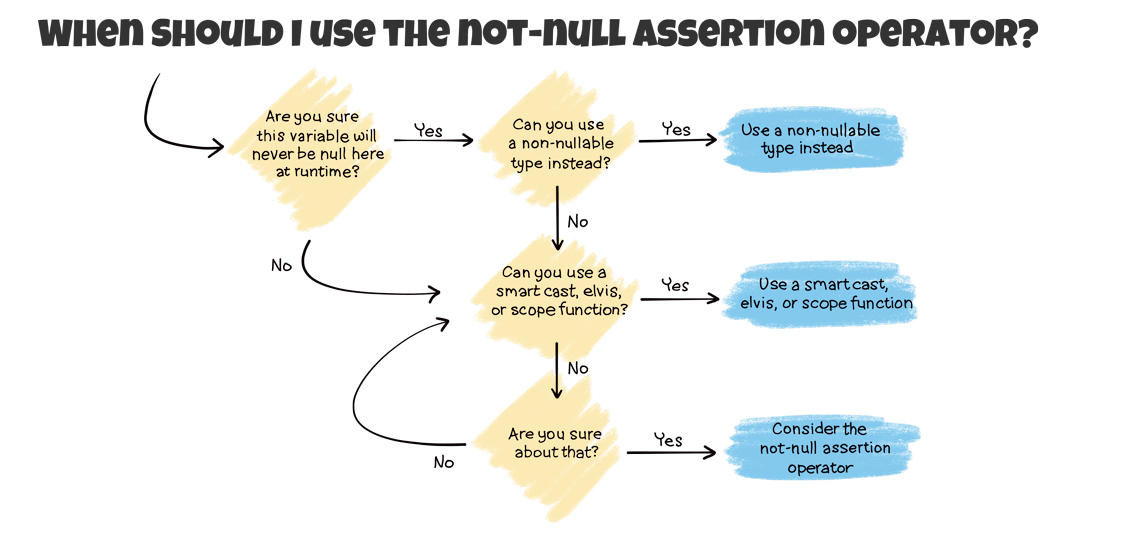
Scope functions, mentioned in the flow chart above, work similarly to a smart cast. We’ll learn about those in a future chapter.
There’s one more null-safety tool that Kotlin gives us. Let’s check it out!
Using the Safe-Call Operator to Invoke Functions and Properties
Back in Chapter 4, we saw how objects have functions and properties, and we can call those by using a dot character. For example, let’s say our Payment
class has a property that tells us what type of payment the customer is using, whether cash, a check, or a card:
In this case, when we get a Payment
, we probably want to do something with it, like print out the type
. Let’s update the orderCoffee()
function to do that.
This works great when the payment
parameter is a non-nullable Payment
type. But when its type is a nullable Payment?
type - as it was when James was running the charity - then we get a compile-time error:
Why is that?
Remember - a variable that has a nullable type - such as Payment?
- is like a bucket that might be empty or might have a value… and we won’t know whether it’s empty until runtime! If the bucket is indeed empty while the code is running, then there would be no actual payment to get the type
from.
In other words, if the payment
isn’t there, then neither is a payment type
! It’s not safe to get the type unless we know that the value of payment
is present. Thankfully, Kotlin gives us a compile-time error, forcing us to deal with this fact.
In this chapter, we’ve learned a few tricks that could help us. For example, we could use an if
to do a smart cast, like this:
When this code runs, if payment
is null, we’ll print “Thank you for supporting us with your encouragement”. Otherwise, if payment
’s value is present, then the message will be based on the type
of payment, such as “Thank you for supporting us with your cash”.
This certainly works, but it’s a lot of code to write. Kotlin provides us with a safe-call operator ?.
that can do the same thing, just more concisely. We can use it along with the elvis operator to achieve the same thing as Listing 6.30, like this:
So, how does the safe-call operator work?
When we look at payment.type.name.lowercase()
, it kind of looks like a train.3
The main change in Listing 6.31 is that we replaced the train car connectors - where we previously used a dot .
, we now use a safe-call operator ?.
:
When Kotlin evaluates a “train” expression like this, you can imagine that it’s hopping from car to car, left to right. When the next connector is a safe-call operator ?.
, it asks, “does this car’s expression evaluate to null?” If so, then it hops off the train with a null
.
Otherwise, it hops to the next car, repeating the process until it finds a null or jumps off the caboose with the final value.
Generally, when writing a train expression, if one of the cars has a nullable type, the rest of the train connectors after it will need to be safe-call operators rather than just dot operators. (I did say generally - we’ll see an exception to this when we get to the chapter about extension functions and properties!)
Summary
Congratulations! You’ve learned a ton in this chapter, including:
- The difference between present and absent values
- The difference between nullable and non-nullable types
- The difference between compile time and runtime
- How to use conditionals to check for nulls
- How to use the elvis operator to provide a default value
- How to use the not-null assertion operator to insist that a value is present
- How to use the safe-call operator to invoke functions and properties on a variable that’s nullable
Proper handling of nulls is an essential skill for every great Kotlin programmer. In the next chapter, we’ll learn about another essential concept - lambdas. See you then!
Thanks to Louis CAD and James Lorenzen for reviewing this chapter.
-
In some Latin-based languages, the word “null” is more closely related to the number zero, but in English it more often refers to something that has no value or effect. When you see it in Kotlin, don’t think of it as the number zero; think of it as “not having a value”. ↩︎
-
Technically, even
null
itself can be considered a value. After all, in real life, even an empty bucket is technically full of air. So, you might hear someone say, “The value of that variable is null,” and that’s fine. However, since it’s easier to learn with the relatable concepts of “present” and “absent”, in this article we’ll regardnull
as the absence of a value rather than as a value itself. ↩︎ -
In fact, the term “train wreck” has been used to describe expressions like this that have many function or property calls chained together. In this book, I won’t add commentary about the advantages or disadvantages of expressions like these. So, instead of calling it a train wreck, I’ll just call it a train! ↩︎